The Power of PDO in Modern Web Development
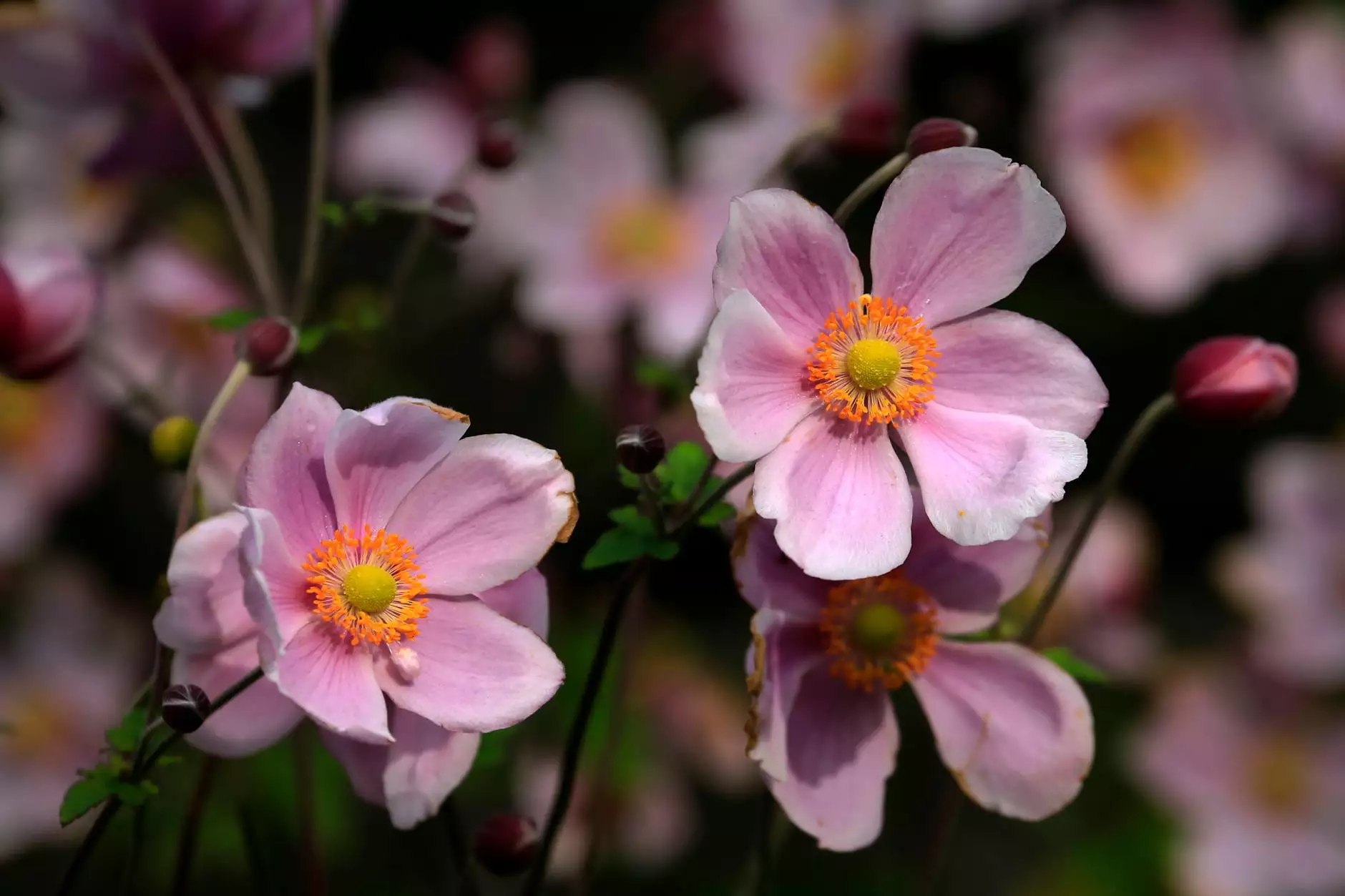
PHP Data Objects (PDO) is a powerful feature within the PHP programming language that enables developers to interact with databases in a secure and efficient manner. In this comprehensive guide, we delve into the significance of PDO, its core functionalities, and how it can be effectively implemented in various business contexts, including dental practices like Swanson Dental.
Understanding PDO: What is it?
PDO, or PHP Data Objects, is an abstraction layer for database access in PHP. It allows developers to work with multiple databases using a consistent API. This means that whether you are using MySQL, PostgreSQL, SQLite, or another database, PDO provides a unified way to execute queries, manage transactions, and handle errors.
Benefits of Using PDO
- Database Independence: With PDO, developers can switch databases without needing to change their code significantly.
- Prepared Statements: PDO supports prepared statements, which protect against SQL injection—one of the most common security vulnerabilities.
- Flexible Error Handling: PDO provides robust error handling options, allowing developers to manage exceptions easily.
- Transaction Support: With transaction management built into PDO, developers can ensure data integrity during complex operations.
Starting with PDO
To begin utilizing PDO, the initial step is to establish a connection to your database. This can be accomplished with the following code snippet:
// Database configuration $host = 'localhost'; $db = 'your_database'; $user = 'your_username'; $pass = 'your_password'; $charset = 'utf8mb4'; // Data Source Name $dsn = "mysql:host=$host;dbname=$db;charset=$charset"; $options = [ PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION, PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC, PDO::ATTR_EMULATE_PREPARES => false, ]; try { $pdo = new PDO($dsn, $user, $pass, $options); } catch (\PDOException $e) { throw new \PDOException($e->getMessage(), (int)$e->getCode()); }Executing Queries with PDO
Once a connection is established, executing queries becomes straightforward. Here’s how to do it using prepared statements, which are essential for security.
Using Prepared Statements
Prepared statements are a technique used in SQL to execute similar queries multiple times efficiently and securely. Here’s an example:
$sql = "SELECT * FROM dentists WHERE id = :id"; $stmt = $pdo->prepare($sql); $stmt->execute(['id' => 1]); $dentist = $stmt->fetch();In the example above, the SQL query selects a dentist’s information based on their ID, using a placeholder :id. The execute method efficiently replaces this placeholder with the specified value, significantly enhancing the security of the application.
Advanced PDO Techniques
Beyond basic query execution, PDO also supports advanced techniques that can enhance your application’s functionality.
Transactions in PDO
Using transactions ensures that a series of database operations are completed successfully before committing them. Here’s a basic example:
try { $pdo->beginTransaction(); // Execute multiple statements $stmt1 = $pdo->prepare("INSERT INTO dentists (name, specialty) VALUES (?, ?)"); $stmt1->execute(['Dr. Smith', 'Cosmetic Dentistry']); $stmt2 = $pdo->prepare("INSERT INTO patients (name, dentist_id) VALUES (?, ?)"); $stmt2->execute(['John Doe', $pdo->lastInsertId()]); // Commit the transaction $pdo->commit(); } catch (Exception $e) { $pdo->rollBack(); echo "Failed: " . $e->getMessage(); }Real-World Applications of PDO in Business
Businesses, such as Swanson Dental, can greatly benefit from implementing PDO in their web applications. Here are a few examples of how PDO enhances business operations:
1. Secure Patient Data Management
In the realm of dental practices, handling sensitive patient information is crucial. By using PDO’s prepared statements, practices can protect this information from unauthorized access and SQL injection attacks, ensuring compliance with data protection regulations.
2. Efficient Appointment Scheduling
Utilizing PDO allows for dynamic appointment scheduling. With PDO, practices can easily add, update, or delete appointment records while maintaining database integrity through transaction management.
3. Personalization and Customization
Businesses can leverage PDO to collect and analyze patient data to offer personalized services. By utilizing PDO’s smooth integration with various databases, practices can create a tailored experience for their patients.
Best Practices for Using PDO
To maximize the potential of PDO in your applications, consider the following best practices:
- Use Prepared Statements: Always opt for prepared statements to execute queries. This is one of the most effective ways to prevent SQL injection threats.
- Handle Exceptions Properly: Implement robust exception handling around your database operations to manage errors gracefully.
- Secure Database Credentials: Always keep your database credentials secure and do not hard-code them in your scripts.
- Keep Your PDO Version Updated: Regularly update your PHP version to exploit the latest features and security enhancements of PDO.
Conclusion
In conclusion, PDO is an essential aspect of modern web development in PHP, offering a secure and efficient way to interact with various databases. Businesses, especially in fields like dentistry, can leverage PDO to create more robust applications that enhance customer experience and streamline operations. By adopting PDO, you empower your applications to handle critical data securely, ensuring a solid foundation for your business’s growth.
Whether you are creating a dental practice website or managing patient records, embracing the power of PDO is an investment in your application’s security and performance. To learn more about the benefits of implementing PDO in your next PHP project, consider consulting with experienced developers or resources dedicated to advanced PHP techniques.
pdo pdo :: param_str 0934225077